How to Join strings – Java8
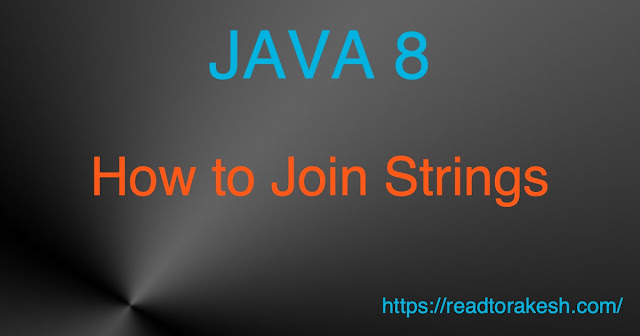
As a programmer quite often we hit the need to join multiple string values separated by a delimiter. For example join all values from a String[] or List separated by comma ( , ). It’s very common scenario and I am sure most of have already done this. Lets how it’s usually done before Java 8 and what is smart way to Join multiple strings in Java 8. We have following array of strings and we need to join all string values from this array into single string separated by pipe ( | ) character String[] quote = {"Java8", "is", "awesome"}; Before Java 8 Before Java 8 usual way to do is, loop through each element of the array and append to another String variable and append separator after each element. May be you are already thinking about general issue with this approach which is unnecessary separator after the last element. To deal with it, either we can add condition within loop to not append separator after last element or remove the last character (